Создание логотипа Mitsubishi с помощью Blender Python API. Устанавливается плагин, как обычно из архива: mitsubishi_logo_blender_python.zip (6.0 КБ) На N-панели должна появится вкладка с кнопкой “Create Logo”
За основу взят пример из туториала:
Я экспортировал в Collada (.dae) формат. Импортировал в C# OpenGL 3 с помощью XPath. Окно создаётся из консольного проекта с помощью библиотеки OpenTK, которая ставится через NuGet. Порт уроков из learnopengl.com на OpenTK на официальном сайте: https://opentk.net/learn/index.html
Путь загрузки модели: “Assets/Models/Logo.dae”. Модель логотипа в формате Collada (.dae): Logo.zip (979 Байт)
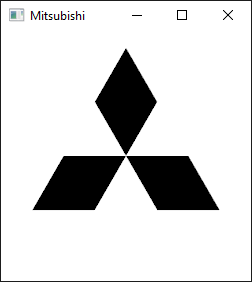
Program.cs
using System;
using OpenTK.Graphics.OpenGL;
using OpenTK.Graphics;
using OpenTK;
using System.Xml;
namespace MitsubishiLogoFromDae
{
class Program
{
static void Main(string[] args)
{
using (var window = new Window())
{
window.Title = "Mitsubishi";
window.Run();
}
}
}
class Window : GameWindow
{
private Matrix4 _projMatrix;
private Matrix4 _modelMatrix;
private Matrix4 _mpMatrix;
private int _uMPMatrixLocation;
private int _amountOfVertices = 0;
public Window() : base(250, 250, new GraphicsMode(32, 0, 0, 8)) { }
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
var vShaderSource =
@"
#version 130
in vec3 aPosition;
uniform mat4 uMPMatrix;
void main()
{
gl_Position = uMPMatrix * vec4(aPosition, 1.0);
}
";
var fShaderSource =
@"
#version 130
precision mediump float;
out vec4 fragColor;
void main()
{
fragColor = vec4(0.0);
}
";
var vShader = GL.CreateShader(ShaderType.VertexShader);
GL.ShaderSource(vShader, vShaderSource);
GL.CompileShader(vShader);
var fShader = GL.CreateShader(ShaderType.FragmentShader);
GL.ShaderSource(fShader, fShaderSource);
GL.CompileShader(fShader);
var program = GL.CreateProgram();
GL.AttachShader(program, vShader);
GL.AttachShader(program, fShader);
GL.LinkProgram(program);
GL.UseProgram(program);
int vbo;
GL.CreateBuffers(1, out vbo);
float[] positions;
LoadData("Assets/Models/Logo.dae", out positions);
_amountOfVertices = positions.Length / 3;
GL.BindBuffer(BufferTarget.ArrayBuffer, vbo);
GL.BufferData(BufferTarget.ArrayBuffer, sizeof(float) * positions.Length, positions, BufferUsageHint.StaticDraw);
var aPositionLocation = GL.GetAttribLocation(program, "aPosition");
GL.VertexAttribPointer(aPositionLocation, 3, VertexAttribPointerType.Float, false, 0, 0);
GL.EnableVertexAttribArray(aPositionLocation);
_uMPMatrixLocation = GL.GetUniformLocation(program, "uMPMatrix");
GL.ClearColor(1f, 1f, 1f, 1f);
}
protected override void OnRenderFrame(FrameEventArgs e)
{
base.OnRenderFrame(e);
GL.Clear(ClearBufferMask.ColorBufferBit);
_modelMatrix =
Matrix4.CreateScale(5f, 5f, 1f) *
Matrix4.CreateTranslation(0f, 0f, -1f);
_mpMatrix = _modelMatrix * _projMatrix;
GL.UniformMatrix4(_uMPMatrixLocation, false, ref _mpMatrix);
GL.DrawArrays(PrimitiveType.Triangles, 0, _amountOfVertices);
SwapBuffers();
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
GL.Viewport(0, 0, Width, Height);
float aspect = (float)Width / Height;
float worldWidth = aspect * 20f;
_projMatrix = Matrix4.CreateOrthographic(worldWidth, 20f, 100f, -100f);
}
private void LoadData(string path, out float[] vertices)
{
XmlDocument xml = new XmlDocument();
xml.Load(path);
XmlNamespaceManager xnm = new XmlNamespaceManager(xml.NameTable);
xnm.AddNamespace("a", "http://www.collada.org/2005/11/COLLADASchema");
XmlElement root = xml.DocumentElement;
XmlNode pNode = root.SelectSingleNode("//a:p", xnm);
int[] p = Array.ConvertAll(pNode.InnerText.Split(new char[] { ' ' }), int.Parse);
XmlNode posNode = root.SelectSingleNode("//a:float_array[substring(@id, string-length(@id) - string-length('mesh-positions-array') + 1) = 'mesh-positions-array']", xnm);
float[] positions = Array.ConvertAll(posNode.InnerText.Split(new char[] { ' ' }), float.Parse);
vertices = new float[3 * p.Length / 2];
int triangleIndex = 0;
for (int i = 0; i < p.Length; i++)
{
if (i % 2 == 0)
{
vertices[triangleIndex++] = positions[p[i] * 3];
vertices[triangleIndex++] = positions[p[i] * 3 + 1];
vertices[triangleIndex++] = positions[p[i] * 3 + 2];
}
}
}
}
}